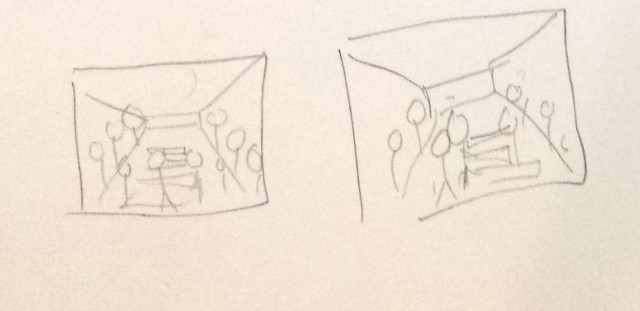
Concept
For my time project I wanted to make a visual clock that showed people crossing the street during the duration of the day. I was inspired by the Beatles album cover Abbey Road and my design was based off that iconic image. I created four figures, each linked to a different time. The smallest, child like figure is milliseconds, the next taller one is seconds which is second fastest across the screen, the fourth fastest figure is minutes. The tallest figure is of course hours. The fastest blue figure was linked to milliseconds, the second fastest figure was linked to seconds, the third, minutes and the last, hours. Each figure would move real-time according to minutes and hours. I also changed the color of the outfits of the minutes, hours and seconds as they crossed the threshold. The traffic light is also a sort of clock as well. From 1 to 4 it is red, from 5 to 8 green and from 9 to 12 it is yellow.
My main goal was to both create a recognizable scene through processing and link that scene to the passage of time. I was especially focusing on visuals for this project seeing as I hadn’t been happy with the overall appearance of some of my other programs. Overall, I like my results, especially considering the amount of time I spent creating the actual image. If possible I would like to add more aspects to the scene including passing vehicles and the like.
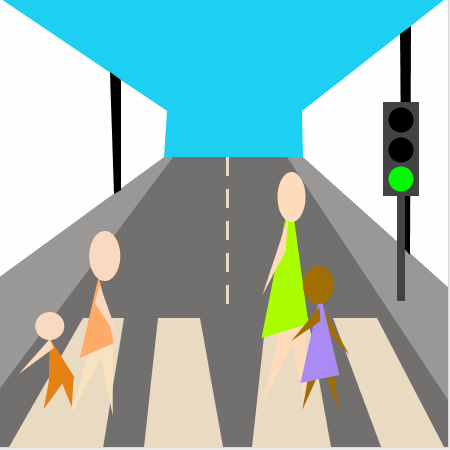
Completed Final version
float skinc=227;
float x=1;
float s= second();
float h= hour();
float m= minute();
float grelight=250;
float grealpha=0;
float relight=0;
float realpha=255;
float yelllight=0;
float yelllightalpha=255;
color milclothesc=color(255,255,255);
float secclothes= 170;
//changing the color of the persons outfit
float change;
float moving3old=0;
void setup(){
size(450,450);
noStroke();
}
void draw(){
smooth();
background(29,208,242);
//street
fill(113,112,111);
beginShape();
vertex(-58,450);
vertex(165,160);
vertex(291,160);
vertex(490,450);
endShape();
//left sidewalk
fill(155,153,151);
beginShape();
vertex(148,160);
vertex(-434,553);
vertex(-63,478);
vertex(175,160);
endShape();
//right sidewalk
fill(155,153,151);
beginShape();
vertex(289,160);
vertex(476,442);
vertex(545,357);
vertex(313,161);
endShape();
fill(255);
//left buildings
beginShape();
vertex(0,0);
vertex(169,114);
vertex(166,160);
vertex(-26,300);
endShape();
//right buildings
beginShape();
vertex(451,-1);
vertex(304,114);
vertex(305,160);
vertex(461,300);
endShape();
fill(0);//gaps between buildings
beginShape();
vertex(112,75);
vertex(123,83);
vertex(123,193);
vertex(116,197);
endShape();
beginShape();//right gaps
vertex(413,29);
vertex(402,37);
vertex(404,249);
vertex(412,258);
endShape();
float time= hour()+minute()+millis();
if(time< =4){//from 0 to 4
grelight=0;
grealpha=255;
relight=250;
realpha=0;
yelllight=0;
yelllightalpha=255;
}
if((time>4)&&(time< =8)){//from 5 to 8 color will be green
grelight=250;
grealpha=0;
relight=0;
realpha=255;
yelllight=0;
yelllightalpha=255;
}
if((time>8)&&(time< =12)){//from 9 to 12it is yellow
grelight=0;
grealpha=255;
relight=0;
realpha=255;
yelllight=250;
yelllightalpha=0;
}
//traffic light
fill(68);
rect(385,105,36,94);
rect(399,188,8,116);
fill(255,0,0,relight);//red light
ellipse(403,123,25,25);
fill(0,0,0,realpha);//not on
ellipse(403,123,25,25);
fill(249,250,91,yelllight);//yellow
ellipse(403,153,25,25);
fill(0,0,0,yelllightalpha);//not on
ellipse(403,153,25,25);
fill(0,255,0,grelight);//green
ellipse(403,182,25,25);
fill(0,0,0,grealpha);//not on
ellipse(403,182,25,25);
noStroke();
for (float i=160; i<320; i=i+32){//creating the peach lines going don the street
fill(234,218,194);
rect(228,i,3,19);
}
fill(234,218,194);//left square
beginShape();
vertex(85, 321);
vertex(126,321);
vertex(91+(-91),1101);
vertex(11,450);
endShape();
fill(234,218,194);//right square
beginShape();
vertex(335, 321);
vertex(379,321);
vertex(875+(-91),1101);
vertex(383,450);
endShape();
for(float e=150; e<289; e=e+108){
fill(234,218,194);//middle square s
beginShape();
vertex(e+10, 321);
vertex(e+52,321);
vertex(e+75,450);
vertex(e+-4,450);
endShape();
}
//mapping the first person with hours
//head4
fill(562,220,187);
float hoursmove= (map(hour(),0,23,0,width));
if( hoursmove<=0){
secclothes= random(0,255);
}
//left leg
triangle(hoursmove+-29,407,hoursmove+12,323,hoursmove+-6,311);
//right leg
triangle(hoursmove+15,416,hoursmove-(-13),307,hoursmove-5,316);
fill(secclothes,544,-2);//stomach color
triangle(hoursmove+(-1),196,hoursmove+-30,341,hoursmove+17,327);//stomach
fill(562,220,187);
ellipse(hoursmove,200,28,50);//head
triangle(hoursmove+-29,298,hoursmove+-3,219,hoursmove+-6,254);//arm top
float minmove= (map(minute(),0,59,0,width));
//head3
if(minmove==0){
secclothes= random(0,255);
}
fill(250,227,192);
//left leg
triangle(minmove+-35,419,minmove+-12,344,minmove+5,344);
//right leg
triangle(minmove+8,419,minmove+-7,344,minmove+8,344);
fill(350,secclothes,104);
triangle(minmove+-6,279,minmove+9,346,minmove+-25,361);//stomach
fill(250,218,192);
ellipse(minmove,259,31,50);//head
//top arm
triangle(minmove+15,344,minmove+-10,306,minmove+-5,288);
//head2
float moving2= millis()%1000;
//actual number of seconds as an whole number
float moving3=round(float(millis()-500)/1000);
float moving= map(moving2,0,1000,0,width);
if(moving3!=moving3old){
milclothesc= color(random(0,255),random(0,255),18);
moving3old = moving3;
}
fill(milclothesc);//stomach color
triangle(moving+0,341,moving-(1),387,moving+25,381);//stomach
//left leg
triangle(moving-6,412,moving-(1),385,moving+18,379);
//right leg
triangle(moving+22,410,moving-(-11),383,moving+24,379);
fill(250,218,192);
//top arm
ellipse(moving,329,29,28);
triangle(moving-31,377,moving-0,342,moving-(-4),351);
//mapping the most left girl in purple with the amount of seconds
float mappingtime= (map(second(), 0,59,0,width));
//changing the color of the persons outfit
if (mappingtime==0){
secclothes= random(0,255);
}if (secclothes>255){
secclothes=0;
}
//head1
fill(160,110,2);
fill(160,110,2);
//back arm
triangle(mappingtime-(-31),358,mappingtime+5,311,mappingtime-3,321);
//back leg
triangle(mappingtime+-16,413,mappingtime-4,346,mappingtime+14,346);
//right leg
triangle(mappingtime+21,417,mappingtime-3,346,mappingtime+13,360);
fill(secclothes,138,245);//stomach color
triangle(mappingtime+2,297,mappingtime-18,386,mappingtime+21,378);//stomach
fill(160,110,2);
//top arm
ellipse(mappingtime,288,31,38);//head
triangle(mappingtime-27,344,mappingtime+2,324,mappingtime-(-1),310);
println("secclothes", secclothes, "moving", moving, "changingtime", mappingtime);
}