CSB — one line —fulcrum
TEN LINES
Move your mouse over the screen. Ten lines will reach out and grab your mouse from an invisible grid of circles. I drew this out in peak condition on paper beforehand, as seen below:
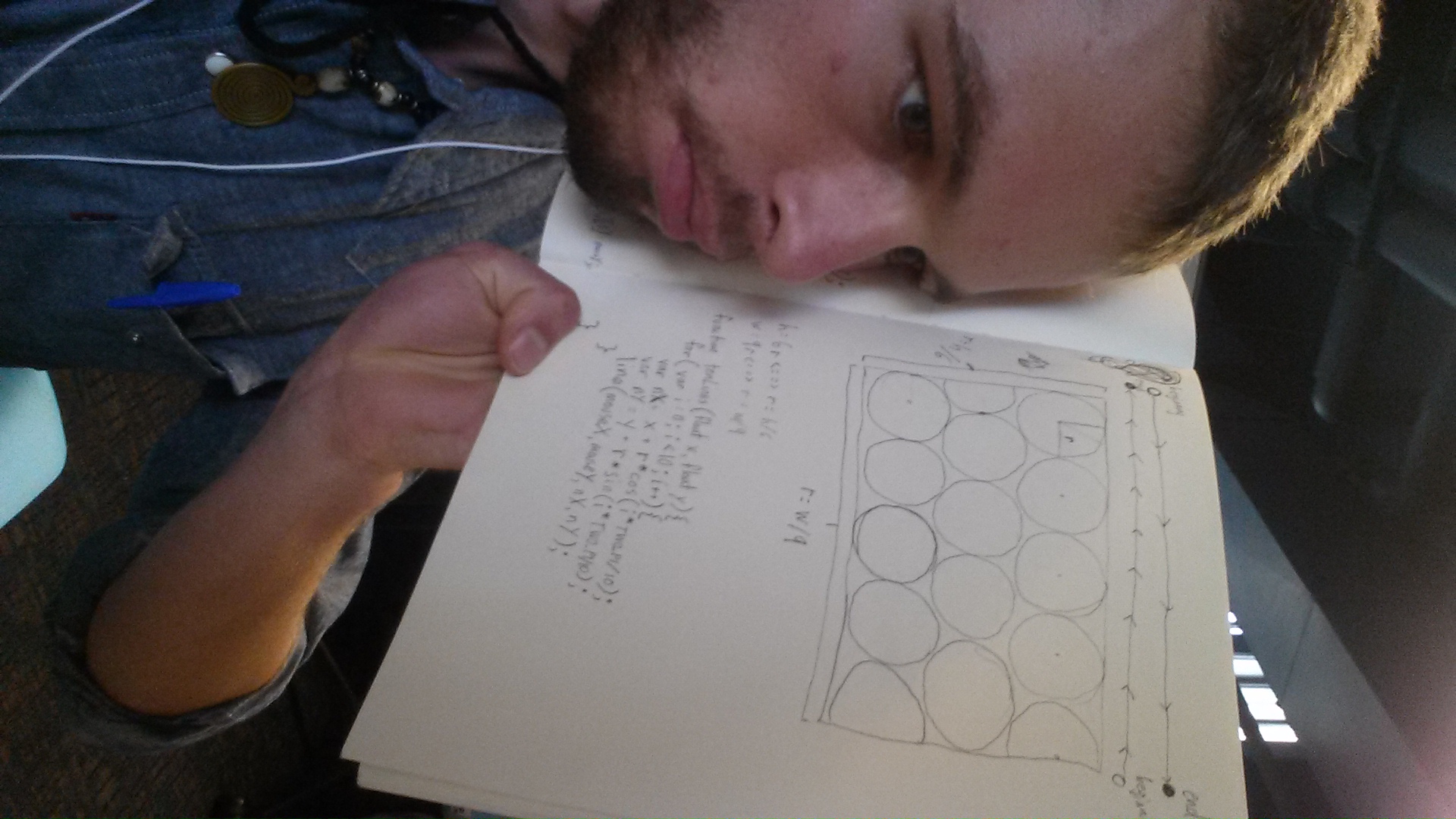
While there were some speed bumps navigating the javascript arrays, I was able to complete it exactly as I had initially envisioned:
One Line : draw one line
There’s not much to say about this. Draw one line, if you try to draw more, pointless operations heat up your processor to punish you for breaking the rules. I came up with this idea while sitting in Concept studio.
Recently, I’ve realized that I haven’t done a true cop out for an assignment in a long time.
1000 Lines: The Red Sea
A sea that is sometimes red, but not often.
this is an ocean build of undulating lines, that slowly change colour with time. Introducing the mouse causes these lines to move, shifting against the movement of the mouse, letting the controller mark their own light trails in the ‘water’.
code:
//The Red Sea. I want to see The Red Sea on a wall.
//class of each light bar that appears onscreen
class Bar{
int ix;
int iy;
int sx;
int sy;
int ex;
int ey;
color c;
Bar(int tempix, int tempiy, int tempsx, int tempsy, int tempex,
int tempey, color tempc){
int rx = int(random(-20,20));
int ry = int(random(-10,10));
ix = tempix;
iy = tempiy;
sx = tempsx + rx;
sy = tempsy + ry;
ex = tempex + rx;
ey = tempey + ry;
c = tempc;
}
//colours are based off of noise and realtive position, so each
//colurs shift in slight uinson.
void update(float t){
c = color(255*noise(ix/20.0,iy/20.0, t/2.0),
255*noise(ix/20.0 + 5,iy/20.0 + 5, t/20.0 + 5),
255*noise(ix/20.0 + 10,iy/20.0 + 10, t/400.0 + 10));
}
//draws with mouse accounted for. Some trig is used to make the
//waves
void display(float t){
stroke(c);
strokeWeight(2);
int sx2 = sx - (mouseX - sx)/4;
int sy2 = sy - (mouseY - sy)/5;
int ex2 = ex - (mouseX - ex)/5;
int ey2 = ey - (mouseY - ey)/6;
line(sx2 + 4*cos(ix/10.0+iy/10.0-t),
sy2 + 10*sin(ix/10.0+iy/10.0-t),
ex2 + 2*cos(ix/10.0+iy/10.0-t),
ey2 + 10*sin(ix/10.0+iy/10.0-t));
}
}
ArrayList<Bar> bars;
//creates the bars semi-randomly
void setup(){
size(1800,500);
bars = new ArrayList<Bar>();
int dw = width/100;
int dh = height/10;
for (int x = 0; x < 100; x++){
for(int y = 0; y < 10; y++){
bars.add(new Bar(x,y,
dw*(x)+int(50*(noise(x/20.0 + 10,y/20.0 + 10)-0.5)),
dh*(y)+int(50*(noise(x/20.0 + 5,y/20.0 + 5)-0.5)),
dw*(x)+5+int(50*noise(x/20.0,y/20.0) - 0.5),
dh*(y)+50+int(50*noise(x/20.0 - 5,y/20.0 - 5)-0.5),
color(255,0,0)));
}
}
noStroke();
fill(0,0,0);
rect(0,0,width,height);
}
//draws with low-alpha background, to allow for blur, and adds any
//time-based trig movement and mouse movement
void draw(){
//background(0,0,0, 200);
noStroke();
fill(0,0,0,3);
rect(0,0,width,height);
float t = millis()/1000.0;
for (int i = bars.size()-1; i >= 0; i--) {
Bar bar = bars.get(i);
bar.update(t);
bar.display(t);
}
}
10 Lines: The Menhirs
Menhirs, also called standing stones, are a type of monolith found in Northern Europe. This is a minimal experiment in landscape generation using these objects as inspiration. They rotate with the mouse, and stones are added and remove by left and right clicking respectively.
code:
//The Menhirs. Ignore the Aztec look.
ArrayList<Menhir> menhirs;
//seperately handles a menhir drawing
void drawMenhir(int x,int y,int dw,int dh){
noStroke();
int ang = dw/4;
fill(255);
beginShape();
vertex(x+dw, y+dh-ang);
vertex(x+dw/2, y+dh);
vertex(x, y+dh-ang);
vertex(x, y+ang);
vertex(x+dw/2,y);
vertex(x+dw,y+ang);
endShape();
stroke(0);
strokeWeight(3);
beginShape();
vertex(x+dw,y+ang + 20);
vertex(x+dw, y+dh-ang);
vertex(x+dw/2, y+dh);
vertex(x+dw/2,y+2*ang);
vertex(x+dw,y+ang);
vertex(x+dw/2,y);
vertex(x, y+ang);
vertex(x, y+dh-ang);
endShape();
noFill();
}
//seperately draws the shadow of a menhir
void drawShadow(int x,int y,int dw,int dh){
int ang = dw/4;
stroke(0);
noFill();
beginShape();
vertex(x, y+dh-ang);
vertex(x-dh,y+dh-ang+(dh/2)); //here be logial derails
vertex(x-dh+dw/10,y+dh-ang+(dh/2)+ang/5);
vertex(x + dw/10, y+dh-ang + ang/5);
vertex(x+ (2*dw/10), y+dh-ang +(2*ang/5));
vertex(x-dh+ (2*dw/10),y+dh-ang+(dh/2)+(2*ang/5));
vertex(x-dh+ (3*dw/10),y+dh-ang+(dh/2)+(3*ang/5));
vertex(x+ (3*dw/10), y+dh-ang +(3*ang/5));
vertex(x+ (4*dw/10), y+dh-ang +(4*ang/5));
vertex(x-dh+ (4*dw/10),y+dh-ang+(dh/2)+(4*ang/5));
vertex(x-dh+ (5*dw/10),y+dh-ang+(dh/2)+(5*ang/5));
vertex(x+ (dw/2) - ang , y+dh-ang + 3*ang/2);
endShape();
}
// class that handles the standing stones
class Menhir{
int x;
int y;
int trueY;
int h;
int w;
float radius;
Menhir(){
x = 0;
y = 0;
trueY = 0;
h = int(random(90,height/4));
w = int(random(30,width/8));
radius = map (noise(millis()), 0.0,1.0 , 0.0,0.6);
}
//given an angle relative to the baseline (y = width/2),
//places the menhir on that angle.
void update(float ang){
x = width/2 + int(width*radius * cos(ang));
y = height/2 + int(height*radius * sin(ang));
trueY = y;
if(mouseX < width/2) {
x = width - x;
y = height - y;
}
}
//I'm bad at code.
void displayShadow(){
drawShadow(x,y,w,h);
}
void display(){
drawMenhir(x,y,w,h);
}
}
//creates the environment and the first menhir
void setup(){
size(700,700);
menhirs = new ArrayList<Menhir>();
menhirs.add(new Menhir());
}
//function that sorts the menhirs based on y position, so that
//they can later drawn from front to back.
//Zach Rispoli helped write this.
ArrayList<Menhir> zjrispolsort(ArrayList<Menhir> menhirs){
ArrayList<Menhir> sorted = new ArrayList<Menhir>();
ArrayList<Menhir> temp = new ArrayList<Menhir>();
for(int i = 0; i < menhirs.size(); i++){
temp.add(menhirs.get(i));
}
while(temp.size() > 0) {
int minIndex = 0;
for(int i = 0; i < temp.size(); i++) {
if(temp.get(i).y < temp.get(minIndex).y) {
minIndex = i;
}
}
sorted.add(temp.get(minIndex));
temp.remove(minIndex);
}
return sorted;
}
//finds the relative angle of the mouse, and uses that to find
//the angle that each menhir should hold, then draws them in place
void draw(){
background(255);
float dx = float(mouseX -(width/2));
float dy = float(mouseY -(height/2));
float mrads = atan(dy/dx);
float diff = TWO_PI/float(menhirs.size()+1);
for (int i = 0; i< menhirs.size(); i++) {
Menhir menhir = menhirs.get(i);
float ang = mrads + diff*(i+1);
menhir.update(ang);
}
ArrayList<Menhir> tempMenhirs = zjrispolsort(menhirs);
//shadows rendered seperately so that all menhirs appear in front
//of the other menhirs' shadows
for (int i = 0; i< tempMenhirs.size(); i++) {
Menhir menhir = tempMenhirs.get(i);
menhir.displayShadow();
}
for (int i = 0; i< tempMenhirs.size(); i++) {
Menhir menhir = tempMenhirs.get(i);
menhir.display();
}
}
// left click adds a menhir up to 10, right click removes a menhir
// down to 1
void mousePressed() {
if (mouseButton == LEFT && menhirs.size() < 10){
println("menhir added");
menhirs.add(new Menhir());
} else if (mouseButton == RIGHT && menhirs.size() > 1){
menhirs.remove(0);
}
}
Alex Ten Liner
For this project, I wanted 10 lines to vibrate around the courser. Several challenges that arose were trying to figure out how to make diagonal lines starting at the bottom left and moving to the top right. I still have not figured out how to make the random variable move on the inverted axis. Therefore, unlike thee other lines that run diagonal from top left to bottom right, horizontal and vertical, the diagonal lines that run from bottom left top top right do not vibrate into each other.
void setup() {
size(400,400);
frameRate(10);
}
void draw(){
background(0);
float dx=random (-10, 10);
float dy=random (-12, 12);
float da=random (-15,15);
float db=random (-17,17);
float dc=random (-20,20);
strokeWeight(3);
//diagonal L to R down
stroke(50);
line(0,0, (mouseX-6)+dx,(mouseY-6)+dx);
stroke(150);
line((mouseX+6)+db,(mouseY+6)+db, width,height);
//horizontal
stroke(75);
line(0,height/2, (mouseX-10)+da,(mouseY));
stroke(175);
line((mouseX+10)+dc,(mouseY), width,height/2);
//vertical
stroke(255);
line(width/2,0, (mouseX),(mouseY-10)+dy);
stroke(125);
line((mouseX),(mouseY+10)+dc, width/2,height);
//diagonal L to R up top
stroke(50);
line(0,350, (mouseX-8)+db,(mouseY+5)+db);
stroke(225);
line((mouseX+5)+dy,(mouseY-8)+dy, 350,0);
//diagonal L to R up btm
stroke(100);
line(50,height, (mouseX-5)+dc,(mouseY+8)+dc);
stroke(200);
line((mouseX+8)+da,(mouseY-5)+da, width,50);
}
1 Line: Blowout
Two shots of a starting generation.
This is a simple test piece involving the reaction of a single line to the mouse. The line in randomly generated, and the points used to construct the curve will move away from the mouse to warp the shape they create.
code:
//Blowout. When you're swirly and you're curly, then you're kitsch
int[] points;
int[] mpoints;
//generates the points used to make the base curve, as well as define
//the static edge cases for the rendered lines
void setup(){
size(600,600);
points = new int[24];
points[0] = 0;
points[1] = 0;
points[22] = width;
points[23] = height;
for(int i = 0; i < 10;i++){
points[2*(i+1)] = int(width * noise((2*i)/1.0));
points[(2*(i+1)+1)] = int(height * noise((2*i + 20)/1.0));
}
for(int i = 0;i<12;i++){
println("point "+i+": "+points[2*i]+", "+points[(2*i)+1]);
}
mpoints = new int[24];
mpoints[0] = 0;
mpoints[1] = 0;
mpoints[22] = width;
mpoints[23] = height;
}
//adjusts the rendered point based on the position of the mouse.
int[] adjust( int x, int y, int px, int py){
int[] result = new int[2];
float dist = sqrt(sq(px-x) + sq(py-y))/60.0;
if(dist == 0.0){ dist = 0.1;}
result[0] = int(px + (px - x)/dist);
result[1] = int(py + (py - y)/dist);
return result;
}
int[] pair;
//creates an updated version of each point based on the original
//generation and the position of the mouse, then draws the line
void draw(){
background(255);
int x = mouseX;
int y = mouseY;
for(int i = 0; i < 10 ; i++){
pair = adjust(x, y, points[2*(i+1)], points[(2*(i+1))+1]);
mpoints[2*(i+1)] = pair[0];
mpoints[(2*(i+1))+1] = pair[1];
}
strokeWeight(3);
noFill();
stroke(0,0,0);
curve(mpoints[0],mpoints[1], mpoints[0],mpoints[1],
mpoints[2],mpoints[3], mpoints[4],mpoints[5]);
for(int i = 0;i<9;i++){
curve(mpoints[2*i],mpoints[2*i+1], mpoints[2*i+2],mpoints[2*i+3],
mpoints[2*i+4],mpoints[2*i+5],mpoints[2*i+6],mpoints[2*i+7]);
}
curve(mpoints[18],mpoints[19], mpoints[20],mpoints[21],
mpoints[22],mpoints[23], mpoints[22],mpoints[23]);
}
1000 lines
This was an edit of my hallway/10 lines. I was hoping to use the camera function in this one, but was unsuccessful in getting it to work.
float winX;
float lineCol, backgroundCol;
float startPoint;
float winSpeed;
void setup() {
size(700, 700, P3D);
winX= width/7;
lineCol=0;
backgroundCol=255;
startPoint=-2500;
}
void draw() {
noStroke();
background(backgroundCol);
if (winSpeed > 5300){
winSpeed=startPoint;
}
winSpeed=winSpeed+20;
for (int h=1; h < 125 ;h++)
{
moveZ(winX-(h*2), height/2, startPoint+h*2, winSpeed+sqrt(h));
}
if (pmouseX < mouseX && winSpeed > 3000 && winSpeed < 3400 ){
strokeWeight(sqrt(winSpeed/100));
if( backgroundCol == 255 )
while (backgroundCol > 0 )
{backgroundCol= backgroundCol-10;
}
if( backgroundCol == 0 )
while (backgroundCol < 255 )
{backgroundCol= backgroundCol+10;
}
if( lineCol == 255 )
while (lineCol > 0 )
{lineCol= lineCol-10;
}
if( lineCol == 0 )
while (lineCol < 255 )
{lineCol= lineCol+10;
}
}
}
void moveZ(float x, float y, float z, float speed) {
z = z + speed;
drawDoors(x*2, y, z);//left door
drawDoors(x*5, y, z+50);//rightdoor
}
void drawDoors(float x, float y, float z) {
float speed=10;
float side = 103;
stroke(lineCol);
strokeWeight(winSpeed);
line(x, y+100, z+50, x, y+100, z-50);
line(x, y+side, z-50, x, y-side, z-50);
line(x, y-100, z-50, x, y-100, z+50);
line(x, y-side, z+50, x, y+side, z+50);
// beginCamera();
// camera(x,y,z+speed,x,y,z+speed, 0, 1, 0);
// endCamera();
}
Clock: The Whoring Hours
A screen shot taken around midday.
The work day in Amsterdam’s Red Light District runs from about 7pm till 3am. This is a sped-up render of a visual clock representing that work period across the world. The image consists of 24 bars, that can be turning on, on or off. Each bar represents a time zone, and there is a small cross on the bar that shares the time zone of the clock (in this case the US east coast). It’s intended as a reminder of what is currently happening across the world, either near or far, and the stories of the people involved. This is a rendering of the change across time sped up:
code:
//The Whoring Hours. This might belong in a sports bar.
// function that handles the drawing of the light. Could be kept
// within the bar class
void drawBar( int sx, int sy, int ex, int ey, float dx,
color lowC, color highC){
int lr = int(red(lowC));
int lg = int(green(lowC));
int lb = int(blue(lowC));
int dr = int(red(highC)) - lr;
int dg = int(green(highC)) - lg;
int db = int(blue(highC)) - lb;
stroke(lr,lg,lb, int(100*dx));
strokeWeight(40);
line(sx,sy,ex,ey);
stroke(lr + dr/3,lg + dg/3,lb + db/3, int(150*dx));
strokeWeight(20);
line(sx,sy,ex,ey);
stroke(lr + int(dx*2*dr/3),lg + int(dx*2*dg/3),
lb + int(dx*2*db/3),int(200*dx));
strokeWeight(7);
line(sx,sy,ex,ey);
stroke(lr + int(dr*dx*dx),lg + int(dg*dx*dx),
lb + int(db*dx*dx),int(255*dx*dx));
strokeWeight(2);
line(sx,sy,ex,ey);
fill(0);
noStroke();
rect(sx-20,sy-40,40,40);
rect(ex-20,ey,40,40);
}
//class for each of the light bars that represent a time zone
class Light {
String name;
int diff;
int sStart;
int hStart;
int sEnd;
int hEnd;
int x;
int y;
//int alpha;
//int r;
float dl;
Light( String tempName, int tempDiff, int tempSS, int tempHS,
int tempSE, int tempHE, int tempX, int tempY){
name = tempName;
diff = tempDiff;
sStart = tempSS;
hStart = tempHS;
sEnd = tempSE;
hEnd = tempHE;
x = tempX;
y = tempY;
dl = 0.0;
}
//gets the relative brightness (dh) based on time of day and
//time difference
void update(int h, float dh){
int nh = (h + diff) % 24;
if ((nh >= hEnd) && (nh < sStart)){
dl = 0.0;
}
else if (nh >= hStart || nh < sEnd){
dl = 1.0;
}
else if (nh == sStart){
dl = dh;
}
else {
dl = 1-dh;
}
}
void display(){
drawBar( x,y,x,y+200, dl, color(255,0,0),color(255,255,255));
textAlign(CENTER);
fill(160,190,190);
text(name,x,y+220);
}
}
ArrayList<Light> lights;
void setup(){
size(1200,300);
lights = new ArrayList<Light>();
for( int i = -4; i<20; i ++){
lights.add(new Light("", i, 19,20, 2,3, (i+5)*width/25,50));
}
}
void draw(){
int s = second();
int m = minute();
int h = hour();
float dh = float(m)/60 + float(s)/(60*60);
background(10);
for (int i = lights.size()-1; i >= 0; i--) {
Light light = lights.get(i);
light.update(h,dh);
light.display();
}
fill(200,200,200);
textAlign(LEFT);
textSize(15);
text("The Whoring Hours:",15,30);
stroke(200,200,200);
strokeWeight(1);
line((5*width/25)-5,270,(5*width/25)+5,270);
line((5*width/25),265,(5*width/25),275);
}