In the Cave from Elizabeth Agyemang on Vimeo.
A week ago I was handed a box filled with all sorts of strange gadgets and gizmos, little parts that could be connected to an Aurdino and used to create something, anything. That’s the task I was given to do. After scrumaging through the remaining items, I found myself drawn to two objects. The first was a simple white stick like object connected to a circlular base, a sparkfun potentiometer I learned. The device immediately felt reminiscent to a flash light, the way it twisted and turned in the same smooth and intuitive fashion. It was based on this encounter, that I created my program.
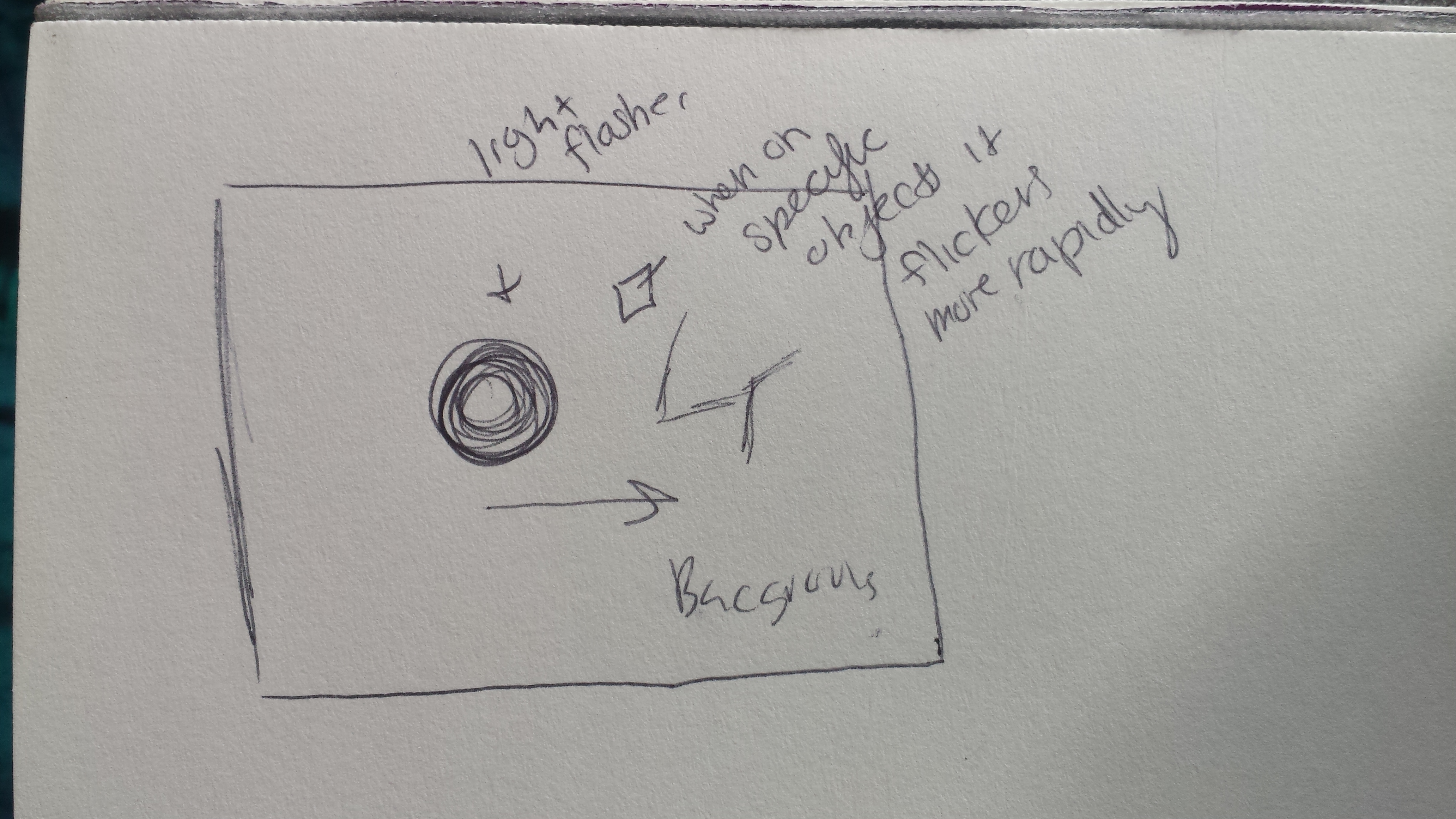
Quick Sketch of the concept
The Cave, is not so much a game as it is an experience, at least, that’s what I envisioned it to be. Though the program is fully functional, including the changing the flashlight size and moving its x position on the screen, their is no audio of dripping water in the cave, no eerie voices or music to set the tone. At least, not yet.
How it Works:
Essentially the player is in control of two functions. You use the large, stick potentiometer to change the size of the flashlight, and the small blue one to shift it on the x-axis.
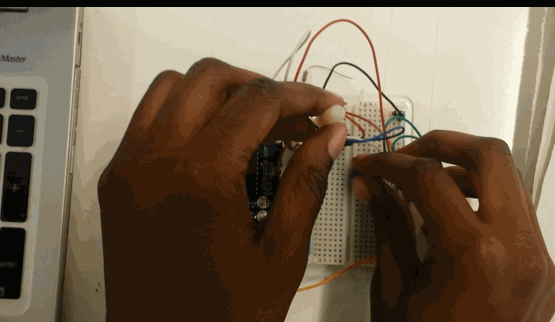
Playing the game
The goal of the game is to find all three clues which you do by pointing the flashlight across the screen. Once a clue is spotted the light will flicker wildly and then the play while be moved on different y-axis of the screen in order to find the next clue.

Problems:
noticed with the sliding potentiometer I was having issues (it kept jumping from value to value randomly), so I switched to another twisting potentiometer which fixed the problem. Also, once the player hits the third clue, I’ve been having issues changing the screen. Hopefully with some more time and coding I’ll be able to fix these issues.
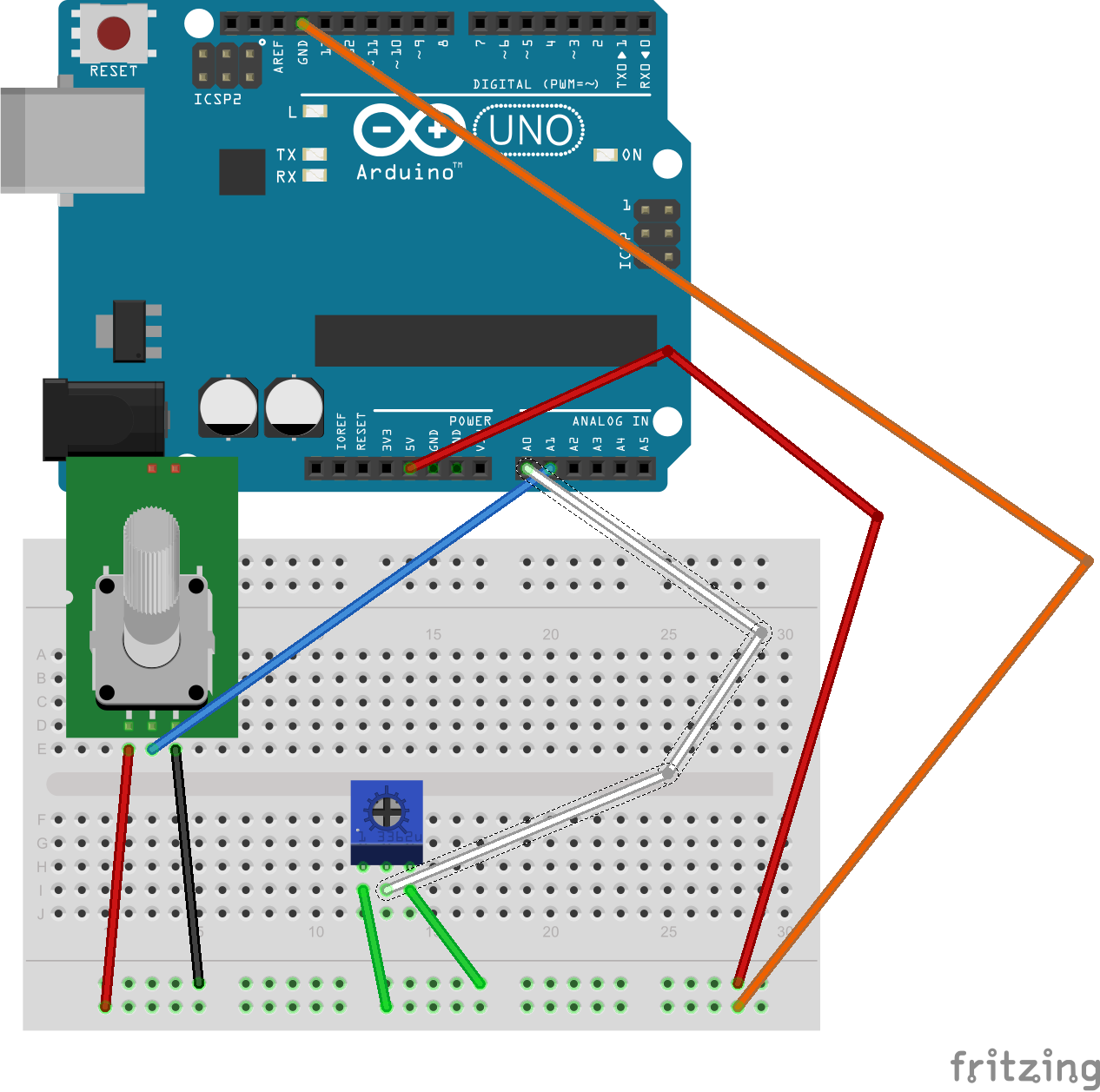
diagram
//game in which you are trying to find 3 instances of murder. Once all three are found the image reveals itself
//background image
PImage backg0;
PImage back1;
PImage back2;
PImage mid3;
PImage mid4;
PImage fro5;
PImage fro6;
PImage fro7;
float lightx;
float lighty;
float opacity=300;
float opacityb=0;
float circ1=100;
float change=0;
//locations of the clues
float clue1x;
float clue2x;
float clue3x;
//blood stains/horror
PImage dripblood;
PImage bottomstain;
PImage hands;
PImage topstain;
PImage lizzie;
PImage crimescene;
float mecirc=50;
//big circle stuff
float bigcx;
float bigcy;
float circlebig=50;
float flashclick=0;
//moving lizzie
float move=753;
float secC=0;
//game counter, counting to see if the images have bee located
//begin at the top, go to middle, then go to the bottom
//load text "Something Happened Here..."
//find the clues
float gamecounter=0;
// This Processing program reads serial data for two sensors,
// presumably digitized by and transmitted from an Arduino.
// It displays two rectangles whose widths are proportional
// to the values 0-1023 received from the Arduino.
// Import the Serial library and create a Serial port handler
import processing.serial.*;
Serial myPort;
// Hey you! Use these variables to do something interesting.
// If you captured them with analog sensors on the arduino,
// They're probably in the range from 0 ... 1023:
int valueA; // Sensor Value A
int valueB; // Sensor Value B
int counter= valueA;
float outcircle;
//distance between flashlight and ci
float d;
float mapB;
void setup(){
size(838,450);
// List my available serial ports
int nPorts = Serial.list().length;
for (int i=0; i < nPorts; i++) {
println("Port " + i + ": " + Serial.list()[i]);
}
// Choose which serial port to fetch data from.
// IMPORTANT: This depends on your computer!!!
// Read the list of ports printed by the code above,
// and try choosing the one like /dev/cu.usbmodem1411
// On my laptop, I'm using port #4, but yours may differ.
String portName = Serial.list()[2];
myPort = new Serial(this, portName, 9600);
serialChars = new ArrayList();
//drawing the background
backg0= loadImage("0Background.png");
back1= loadImage("1back.png");
back2= loadImage("2back.png");
mid4= loadImage("4middle.png");
fro5= loadImage("5front.png");
fro6= loadImage("6front.png");
fro7= loadImage("7fronts.png");
mid3= loadImage("3middle.png");
//drawing the blood stain DONT FORGET BLUR
dripblood= loadImage("blood.png");
bottomstain= loadImage("bottomstain.png");
hands= loadImage("hidhands.png");
topstain= loadImage("top stain.png");
lizzie= loadImage("lizzie2.png");
opacity1= map(d,0,valueA,0,250);
}
float opacity1;
void draw(){
// Process the serial data. This acquires freshest values.
//value of y
lighty=250;
processSerial();
//background
image(backg0,0,0);
image(back1,48,-11);
image(back2,-9,-2);
image(mid3,506,87);
image(mid4,287,257);
image(fro5,0,32);
image(fro7,324,385);
image(fro6,284,146);
//blood stains/horror
image(dripblood,636,-2);
image(bottomstain,-91,31);
image(hands,161,171);
image(topstain,-91,31);
image(lizzie,move,91);
noStroke();
smooth();
float cirx=80;
//mapping
//bigger circles
for(bigcx=11; bigcx<width; bigcx=bigcx+58){
for(bigcy=26; bigcy<height;bigcy=bigcy+47){ //if the distance between the flashlight and the circles is less then 25 and //mapping the opacity to the distance of the light source. as it increases so does the opacity float d= dist(valueB,lighty,bigcx,bigcy); // opacity1= map(d,0,valueA,0,250); // if ((dist(valueB,lighty,bigcx,bigcy)<25)&&(valueA>=cirx)){
// opacity1=0;
//
// }else{
// opacity1=map(d,0,valueA,0,250);
//
// }
if (valueA< =7){ opacity1=248; }else{ opacity1=map(d,0,valueA,0,250); } fill(0,0,0, opacity1);//opacity1 ellipse(bigcx,bigcy,cirx,cirx); // println("opacity1", opacity1, "valB:", valueB, "valA", valueA); //locations of the clues clue1x=248; clue2x=656; clue3x=832; while ((valueB==clue3x)&&(gamecounter>2)){
opacity1=0;
}
}
//if the flashlight is on the clue add to counter and move to next line in image
if((dist(valueB,lighty,clue1x,254)< =5)||(valueB==clue1x)){ secC= random(15); gamecounter=1; }else{ secC=secC; lighty=lighty; } if (gamecounter==1){ lighty=10; } if ((dist(valueB, lighty, clue2x,13)<5)||(valueB==clue2x)&&(gamecounter>=1)&&(lighty==10)){
secC= random(40);
gamecounter=2;
lighty=130;
}
if ((dist(valueB, lighty, clue3x,13)<5)||(lighty==130)||(gamecounter>=2) ){
lighty=130;
secC= random(40);
gamecounter=3;
}if((gamecounter==2)&&(valueB==clue3x)){
gamecounter=3;
opacity1=random(100);
}
if((gamecounter==3)&&(valueB==clue3x)){
move= random(753);
}
}
fill(255,200,0,0);
rect(clue1x,254,5,5);
rect(clue2x,13,5,5);
rect(clue3x,140,5,5);
//flashlight
//change the blue, ie the last number to lighten it
fill(254,255,0,25*1/2*secC);
//the size of the flashlight is being mapped to the mouse
lightx= map(mouseX,0,width,0,850);
// lighty= map(valueB,0,height,0,200);
ellipse(valueB, lighty,flashclick,flashclick);
//main center circle
fill(254,255,0,secC);
ellipse(valueB,lighty,valueA,valueA);
//outside rim
fill(254,255,0,secC*1/2);
outcircle= valueA+(valueA+(valueA*1/2));
ellipse(valueB,lighty, outcircle,outcircle);
//changing the opacity of the flashlight
if (valueA< =7){
secC=0;
counter=valueA;}
while((valueA>counter)){
secCsecC+ 5;
counter=valueA;
}
while (valueA 0) {
char aChar = (char) myPort.read();
// You'll need to add a block like one of these
// if you want to add a 3rd sensor:
if (aChar == 'A') {
bJustBuilt = false;
whichValueToAccum = 0;
} else if (aChar == 'B') {
bJustBuilt = false;
whichValueToAccum = 1;
} else if (((aChar == 13) || (aChar == 10)) && (!bJustBuilt)) {
// If we just received a return or newline character, build the number:
int accum = 0;
int nChars = serialChars.size();
for (int i=0; i < nChars; i++) {int n = (nChars - i) - 1; int aDigit = ((Integer)(serialChars.get(i))).intValue();
accum += aDigit * (int)(pow(10, n)); }
// Set the global variable to the number we captured.
// You'll need to add another block like one of these
// if you want to add a 3rd sensor:
if (whichValueToAccum == 0) {valueA = accum;// println ("A = " + valueA) }
else if (whichValueToAccum == 1) {valueB = accum; // println ("B = " + valueB);}
// Now clear the accumulator
serialChars.clear();bJustBuilt = true;}
else if ((aChar >= 48) && (aChar < = 57)) {
// If the char is between '0' and '9', save it.
int aDigit = (int)(aChar - '0');
serialChars.add(aDigit);
}
}
}
Aurdion code:
// program reads two analog signals from two potentiometers
int sensorValue0 = 0; // variable to store the value coming from the sensor
int sensorValue1 = 0; // variable to store the value coming from the other sensor
void setup() {
Serial.begin(9600); // initialize serial communications
}
void loop() {
// Read the value from the sensor(s):
sensorValue0 = analogRead (A0); // reads value from Analog input 0
sensorValue1 = analogRead (A1); // reads value from Analog input 1
Serial.print ("A");
Serial.println (sensorValue0);
Serial.print ("B");
Serial.println (sensorValue1);
delay (50); // wait a fraction of a second
}
/*
*/