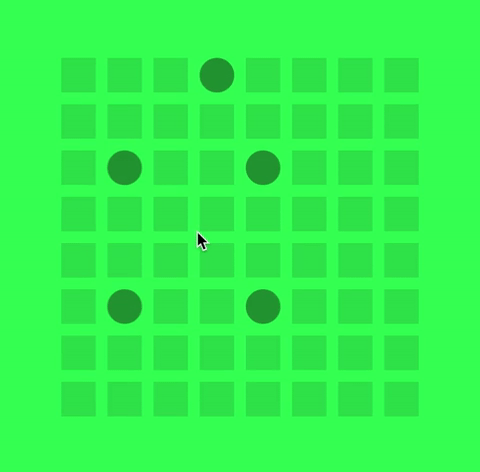
//global values
var boolDoRefresh;
var grid = [];
var gridSize = 8;
var spotSize = 30;
var margin = 20;
var spotGap = 10;
var probabilityOfCircle = .1
function setup() {
createCanvas(400, 400);
boolDoRefresh =true;
//create the first grid
recreateGrid();
//calculate margin based on grid and canvas size
margin = (width - gridSize*(spotSize+spotGap))/2;
}
function draw() {
background(41, 233, 86);
//create a new grid if a mouse was clicked
if(boolDoRefresh) {
recreateGrid();
boolDoRefresh = false;
}
drawGrid();
}
function mousePressed() {
boolDoRefresh = true;
}
function drawGrid(){
for(row = 0; row < gridSize; row++){
for(col = 0; col < gridSize; col++){
grid[row][col].drawSpot();
}
}
}
function spot(x, y, isSq){
this.xPos = x;
this.yPos = y;
this.isSquare = isSq;
this.drawSpot = function(){
if(this.isSquare){
stroke(0, 75);
strokeWeight(0);
fill(0, 0, 0, 30);
rect(this.xPos, this.yPos, spotSize, spotSize);
}else{
stroke(0, 150);
strokeWeight(0);
fill(0, 0, 0, 100);
ellipse(this.xPos + spotSize/2, this.yPos + spotSize/2, spotSize, spotSize);
}
}
}
//recreate the grid, assigning each spot a new shape
function recreateGrid(){
grid = [];
for(row = 0; row < gridSize; row++){
tempRow = [];
for(col = 0; col < gridSize; col++){
newSpot = new spot(margin + col*(spotSize+spotGap), margin + row*(spotSize+spotGap), chooseShape());
tempRow.push(newSpot);
}
grid.push(tempRow);
}
}
//randomly assign a shape based on the give square vs circle probability
function chooseShape(){
var randVal = random(0,1);
if(randVal <= probabilityOfCircle){
return false;
}else{
return true;
}
} |
//global values
var boolDoRefresh;
var grid = [];
var gridSize = 8;
var spotSize = 30;
var margin = 20;
var spotGap = 10;
var probabilityOfCircle = .1
function setup() {
createCanvas(400, 400);
boolDoRefresh =true;
//create the first grid
recreateGrid();
//calculate margin based on grid and canvas size
margin = (width - gridSize*(spotSize+spotGap))/2;
}
function draw() {
background(41, 233, 86);
//create a new grid if a mouse was clicked
if(boolDoRefresh) {
recreateGrid();
boolDoRefresh = false;
}
drawGrid();
}
function mousePressed() {
boolDoRefresh = true;
}
function drawGrid(){
for(row = 0; row < gridSize; row++){
for(col = 0; col < gridSize; col++){
grid[row][col].drawSpot();
}
}
}
function spot(x, y, isSq){
this.xPos = x;
this.yPos = y;
this.isSquare = isSq;
this.drawSpot = function(){
if(this.isSquare){
stroke(0, 75);
strokeWeight(0);
fill(0, 0, 0, 30);
rect(this.xPos, this.yPos, spotSize, spotSize);
}else{
stroke(0, 150);
strokeWeight(0);
fill(0, 0, 0, 100);
ellipse(this.xPos + spotSize/2, this.yPos + spotSize/2, spotSize, spotSize);
}
}
}
//recreate the grid, assigning each spot a new shape
function recreateGrid(){
grid = [];
for(row = 0; row < gridSize; row++){
tempRow = [];
for(col = 0; col < gridSize; col++){
newSpot = new spot(margin + col*(spotSize+spotGap), margin + row*(spotSize+spotGap), chooseShape());
tempRow.push(newSpot);
}
grid.push(tempRow);
}
}
//randomly assign a shape based on the give square vs circle probability
function chooseShape(){
var randVal = random(0,1);
if(randVal <= probabilityOfCircle){
return false;
}else{
return true;
}
}